A successful geolocation request will return a JSON-formatted response defining a location and radius. Location: The user’s estimated latitude and longitude, in degrees. Contains one lat and one lng subfield. Accuracy: The accuracy of the estimated location, in meters. This represents the radius of a circle around the given location. Visual Studio and VS Code extensions.Add-ons that can customize the Visual Studio experience.Add your own items to menus and toolbars.Extend existing tool windows or create your own.Customize IntelliSense for a language or add support for a new one.Create new project templates.
- Vs Studio Mac App Embedded Resource Location Download
- Vs Studio Mac App Embedded Resource Location Chart
- Vs Studio Mac App Embedded Resource Location Software
- Vs Studio Mac App Embedded Resource Locations
This Wiki page explains how to create a simple application using CEF3.
Note to Editors: Changes made to this Wiki page without prior approval via the CEF Forum or Issue Tracker may be lost or reverted.
Technologies to build an app with geolocation. To build a location-based app, we need two components: location services and maps. There are a few different ways to determine outdoor geolocation with various services. We'll talk about the most widespread of them. All modern smartphones equipped with a Global Positioning System chip inside. Code Composer Studio comprises a suite of tools used to develop and debug embedded applications. It includes an optimizing C/C compiler, source code editor, project build environment, debugger, profiler, and many other features. Some antivirus software can interfere with the Android Studio build process, causing builds to run dramatically slower. When you run a build in Android Studio, Gradle compiles your app’s resources and source code and then packages the compiled resources together in an APK. During this process, many files are created on your computer.
- Getting Started
- Source Code
- Build Steps
This tutorial explains how to create a simple application using CEF3. It references the cefsimple example project. For complete CEF3 usage information visit the GeneralUsage Wiki page.
CEF provides a sample project that makes it really easy to get started with CEF development. Simply browse over to the cef-project website and follow the step-by-step instructions. The source files linked from this tutorial are for the current CEF3 master branch and may differ slightly from the versions that are downloaded by cef-project.
Loading a Custom URL
The cefsimple application loads google.com by default but you can change it to load a custom URL instead. The easiest way to load a different URL is via the command-line.
You can also edit the source code in cefsimple/simple_app.cc and recompile the application to load your custom URL by default.
All CEF applications have the following primary components:
- The CEF dynamic library (libcef.dll on Windows, libcef.so on Linux, “Chromium Embedded Framework.framework” on OS X).
- Support files (*.pak and *.bin binary blobs, etc).
- Resources (html/js/css for built-in features, strings, etc).
- Client executable (cefsimple in this example).
The CEF dynamic library, support files and resources will be the same for every CEF-based application. They are included in the Debug/Release or Resources directory of the binary distribution. See the README.txt file included in the binary distribution for details on which of these files are required and which can be safely left out. See below for a detailed description of the required application layout on each platform.
The below list summarizes the items of primary importance for this tutorial:
- CEF uses multiple processes. The main application process is called the “browser” process. Sub-processes will be created for renderers, plugins, GPU, etc.
- On Windows and Linux the same executable can be used for the main process and sub-processes. On OS X you are required to create a separate executable and app bundle for sub-processes.
- Most processes in CEF have multiple threads. CEF provides functions and interfaces for posting tasks between these various threads.
- Some callbacks and functions may only be used in particular processes or on particular threads. Make sure you read the source code comments in the API headers before you begin using a new callback or function for the first time.
Read the GeneralUsage Wiki page for complete discussion of the above points.
The cefsimple application initializes CEF and creates a single popup browser window. The application terminates when all browser windows have been closed. Program flow is as follows:
- The OS executes the browser process entry point function (main or wWinMain).
- The entry point function:
- Creates an instance of SimpleApp which handles process-level callbacks.
- Initializes CEF and runs the CEF message loop.
- After initialization CEF calls SimpleApp::OnContextInitialized(). This method:
- Creates the singleton instance of SimpleHandler.
- Creates a browser window using CefBrowserHost::CreateBrowser().
- All browsers share the SimpleHandler instance which is responsible for customizing browser behavior and handling browser-related callbacks (life span, loading state, title display, etc).
- When a browser window is closed SimpleHandler::OnBeforeClose() is called. When all browser windows have closed the OnBeforeClose implementation quits the CEF message loop to exit the application.
Your binary distribution may include newer versions of the below files. However, the general concepts remain unchanged.
Entry Point Function
Execution begins in the browser process entry point function. This function is responsible for initializing CEF and any OS-related objects. For example, it installs X11 error handlers on Linux and allocates the necessary Cocoa objects on OS X. OS X has a separate entry point function for helper processes.
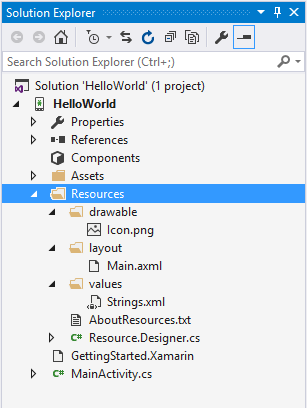
- Windows platform implementation: cefsimple/cefsimple_win.cc
- Linux platform implementation: cefsimple/cefsimple_linux.cc
- Mac OS X platform implementation
- For the browser process: cefsimple/cefsimple_mac.mm
- For sub-processes: cefsimple/process_helper_mac.cc
SimpleApp
SimpleApp is responsible for handling process-level callbacks. It exposes some interfaces/methods that are shared by multiple processes and some that are only called in a particular process. The CefBrowserProcessHandler interface, for example, is only called in the browser process. There’s a separate CefRenderProcessHandler interface (not shown in this example) that is only called in the render process. Note that GetBrowserProcessHandler() must return |this| because SimpleApp implements both CefApp and CefBrowserProcessHandler. See the GeneralUsage Wiki page or API header files for additional information on CefApp and related interfaces.
- Shared implementation: cefsimple/simple_app.h, cefsimple/simple_app.cc
SimpleHandler
SimpleHandler is responsible for handling browser-level callbacks. These callbacks are executed in the browser process. In this example we use the same CefClient instance for all browsers, but your application can use different CefClient instances as appropriate. See the GeneralUsage Wiki page or API header files for additional information on CefClient and related interfaces.
- Shared implementation: cefsimple/simple_handler.h, cefsimple/simple_handler.cc
- Windows platform implementation: cefsimple/simple_handler_win.cc
- Linux platform implementation: cefsimple/simple_handler_linux.cc
- Mac OS X platform implementation: cefsimple/simple_handler_mac.mm
Build steps vary depending on the platform. Explore the CMake files included with the binary distribution for a complete understanding of all required steps. The build steps common to all platforms can generally be summarized as follows:
- Compile the libcef_dll_wrapper static library.
- Compile the application source code files. Link against the libcef dynamic library and the libcef_dll_wrapper static library.
- Copy libraries and resources to the output directory.
Windows Build Steps
- Compile the libcef_dll_wrapper static library.
- Compile/link cefsimple.exe.
- Required source code files include: cefsimple_win.cc, simple_app.cc, simple_handler.cc, simple_handler_win.cc.
- Required link libraries include: comctl32.lib, shlwapi.lib, rcprt4.lib, libcef_dll_wrapper.lib, libcef.lib, cef_sandbox.lib. Note that cef_sandbox.lib (required for sandbox support) is a static library currently built with Visual Studio 2015 Update 3 and it may not compile with other Visual Studio versions. See comments in cefsimple_win.cc for how to disable sandbox support.
- Resource file is cefsimple.rc.
- Manifest files are cefsimple.exe.manifest and compatibility.manifest.
- Copy all files from the Resources directory to the output directory.
- Copy all files from the Debug/Release directory to the output directory.
The resulting directory structure looks like this for 2526 branch:
Linux Build Steps
- Compile the libcef_dll_wrapper static library.
- Compile/link cefsimple.
- Required source code files include: cefsimple_linux.cc, simple_app.cc, simple_handler.cc, simple_handler_linux.cc.
- Required link libraries include: libcef_dll_wrapper.a, libcef.so and dependencies (identified at build time using the “pkg-config” tool).
- Configure the rpath to find libcef.so in the current directory (“-Wl,-rpath,.”) or use the LD_LIBRARY_PATH environment variable.
- Copy all files from the Resources directory to the output directory.
- Copy all files from the Debug/Release directory to the output directory.
- Set SUID permissions on the chrome-sandbox executable to support the sandbox. See binary distribution build output for the necessary command.
The resulting directory structure looks like this for 2526 branch:
Mac OS X Build Steps
- Compile the libcef_dll_wrapper static library.
- Compile/link/package the “cefsimple Helper” app.
- Required source code files include: process_helper_mac.cc.
- Required link frameworks include: AppKit.framework.
- App bundle configuration is provided via “cefsimple/mac/helper-Info.plist”.
- Load the CEF Framework as described here.
- Compile/link/package the “cefsimple” app.
- Required source code files include: cefsimple_mac.mm, simple_app.cc, simple_handler.cc, simple_handler_mac.mm.
- Required link frameworks include: AppKit.framework.
- App bundle configuration is provided via “cefsimple/mac/Info.plist”.
- Load the CEF Framework as described here.
- Create a Contents/Frameworks directory in the cefsimple.app bundle. Copy the following files to that directory: “cefsimple Helper.app”, “Chromium Embedded Framework.framework”.
The resulting directory structure looks like this for 2526 branch:
Updated
The trend of geolocation has densely entrenched in the mobile application market. Geolocation in the mobile app has opened the door for new startup ideas and has established businesses that basically couldn't exist without this technology.
On the other hand, the ability to integrate geolocation in the mobile app has brought a new client service treatment for existing goods and services and a new level of marketing strategies. It has changed the whole workflow of interaction with the consumer.
Some applications are entirely based on this technology, and even created a new niche of location-based services in the market. Others use it as an additional feature to extend the service and make it more advanced.
Here's our take on explaining how to make a location-based app. It is not as easy as it seems since many features are overlapped, complement each other, or extrapolates attributes to other areas, thus creating a new niche. So, please, don't hesitate to share your thoughts in the comments.
How to make a location-based app: step-by-step
Step 1. Research Your Idea
Research the market and competitors to look at the market potential and figure out how to make your app better.
Step 2. Create Wireframe of your geolocation app
To bring your idea into reality, you and your app development team need to put your idea down on paper and develop a storyboard.
Step 3. Develop GPS app MVP
Create a minimum variable product to evaluate your business idea at a minimum budget and receive feedback from app users.
Step 4. Create a feature-rich map-based app
After receiving user feedback, you can start the second development stage and add other important features to your app.
Mobile Apps that Benefit from Geolocation Feature
Maps and navigation
The first thing that comes to mind when we talk about geolocation app development, a service that helps to navigate the terrain. These include Google Maps, Waze, as well as a variety of compasses, schedules of urban transport, and astronomy.
Vs Studio Mac App Embedded Resource Location Download
Read our article about creating a navigation app like Waze to know more.
Place annotation and recommendations Vsmahome app for mac free.
Such apps are all about places to go out and recalls from other consumers. We consistently receive a lot of requests for GPS app development for a startup like Yelp and Foursquare.
[The APP Solutions Project for Nuwbii]
Geosocial apps
This type of apps is more about social components than geolocation. Users share content based on locations in apps like Instagram and Facebook Places. This category also includes streaming services like Periscope.
GPS tracking apps
This type of apps is widely used for security reasons: to keep children protected, to find a stolen car, to keep a check on employees or family members.
Weather forecast applications
Apps like Yahoo Weather and Weather Underground use geolocation data to increase the usability and skip a few steps between the consumer and the product. You have such apps or widgets on your smartphone for sure. There is one more interesting fact about it. Weather apps are the most leading category to which users turn on geolocation. About 65% in comparison to 38% of social networking apps.
On-demand services
The technology of geolocation has enabled a new way to provide such services as taxi, food delivery or domestic issues assistance. Talking about this category, we should mention Uber and Lyft.
Health and fitness apps
Apps that use GPS data for tracking sports activities and patterns. The most famous of them are RunKeeper, Runtastic, and Moves.
Lifestyle and hobbies
Lifestyle apps connect users according to their interests and lifestyle - for example, their passion for golf or hiking.
[The APP Solutions Project for All Square]
How to send a text from your Mac Click on the Messages icon in the Dock at the bottom of the screen (it's a blue speech bubble), or search by pressing Cmd + Space bar and start to type Messages. Set up iMessage. IMessage is the Apple messaging service you get automatically with your Apple. How to get message app icon on mac.
Event apps
Event applications provide the organization of events and put together event hosts and users. One of the biggest players in this market is Eventbrite.
Travel apps
All types of apps that are required to set up a vacation or business trip. Very often they are an extension of the web version. In this niche, you can find TripAdvisor, Airbnb, and a lot of others.
Time-based and memory apps
They use information about the location to remind customers about events that took place in the same spot. Check Path and TimeHop for some magic.
Social networking and dating services
Dating services, along with usual social media apps, help to connect people according to their business or private interests. The niche of dating services like Tinder or Badoo has reached a massive part of the market recently.
Local experts and real-time knowledge
Apps like Localmind or Nextdoor help to get people together in their neighborhood for social and political initiatives and connect people with local experts. Outlook for mac auto address fill says otherwise.
[The APP Solutions Project for Que Pro]
E-commerce apps
Mobile apps for electronic commerce business like eBay or Asos make the everyday consumer experience more fast and smart, and information about geographical location brings new advantages for logistics and delivery.

Vs Studio Mac App Embedded Resource Location Chart
For more details also check 'Do You Need a Mobile eCommerce App for Your Business?'
Apps for offline business
Even offline businesses can get a profit from the location-based mobile app for its users. Firstly, it’s an easy way to inform customers and provide a loyalty program. Secondly, there are indoor location technologies, which are just intended for offline stores. About it read a bit below.
Augmented reality and games
2020 seems to be a year of augmented/virtual realities. The popular brands lightning-fast picked up this trend for their advertising campaigns and a feature of geolocation makes this experience more bright and complete. Same thing with the games.
Technologies to build an app with geolocation
To build a location-based app, we need two components: location services and maps.
There are a few different ways to determine outdoor geolocation with various services. We'll talk about the most widespread of them.
GPS
All modern smartphones equipped with a Global Positioning System chip inside. GPS uses the information about the location and timing that satellites send from space. The smartphone needs data from at least four satellites to determine position with about 60 feet accuracy.
Cell ID
If the GPS signal is unavailable, we can use the information from cell towers. Mobile networks help to determine which cell uses the customer and compare it with a base-stations database. This method works better in major cities with a vast amount of cells.
Assisted GPS
An assisted GPS approach combines GPS and Cell ID tools and happens to be even more precise than just GPS by itself.
Wi-Fi
Wi-Fi determines the position of the user the same way the Cell ID does, but it is more precise because it covers smaller areas.
There two ways to determine location with Wi-Fi. The first one is RSSI (receive a signal strength indication) that refers to signals from the phone with Wi-Fi points database. The other one is used infrequently visited places. It uses profiles of some locations that are on Wi-Fi networks and called a wireless fingerprint. It identifies the user's position with 2 meters accuracy.
After the location of a user is captured, we use services like Google Maps geolocation or some Map Kit Framework to put it on a real map. Google Maps APIs for iOS and Android devices provide the performance of geolocation apps, including all information about the location, detailed maps, search for nearby places, and other features.
Google Maps SDK for iOS is responsible for adding maps and Google Places API for iOS implements the detection of location and other advanced features. The same tools are provided for Android devices with Google Maps Android API and Google Places API for Android.
Vs Studio Mac App Embedded Resource Location Software
Our developers usually recommend building native applications, which means custom development for every single operating system. But there is also an option to create a cross-platform app using HTML5 geolocation and JavaScript geolocation libraries.
Indoor Geolocation Technologies
Speaking of marketing facilities of geolocation, we also should mention indoor technologies of Geofencing and iBeacon/Eddystone. Here are two interesting facts that will help you to do justice to these tools.
Firstly, according to studies, the indoor location-based market has grown from $8,12 billion in 2014 to $30,6 billion in 2016. Secondly, some mobile devices per capita are on the rise from 9.6% in 2011 to 31.3% in 2016. To top it all, it is worth noting that 8 of 10 consumers prefer using their smartphones for making a final decision before purchasing in the market.
All this indicates that location-based marketing is the new step to customer acquisition and retention, so don't miss it while planning your next marketing strategy.
Geofencing
Geofencing uses GPS in users' mobile devices to determine how close they are to a particular point: whether users are inside or outside the shop or they have just entered or left it. Although geofencing can be used as a way of sending offers or coupons to consumers, the operating range is much further and can be used to provide a personalized experience.
There are three types of geofencing triggers:
- Static triggers are based on the user's position in a particular place. For example, a message comes to individual users when they enter the store.
- Dynamic triggers are based on the user's location and let them know about the changing data stream. For example, a notification about the free parking space is sent to the user that is passing nearby.
- The combined approach is based on the user's location about other users. For example, notifications about friend's check-in with apps like Yelp, Facebook, or Foursquare.
iBeacon and Eddystone
Apple iBeacon & Google Eddystone technologies do not deliver offers or other content by themselves. They only send out the identifiers (Bluetooth Low Energy signals, BLE), which trigger actions in custom-designed mobile apps for iBeacon/Eddystone.
Unlike geofencing, beacons cannot determine the user's position on the map. Instead of this, they use BLE to assess whether the mobile user is in the area of their performance.
Geofencing is much less accurate when it is necessary to determine the precise geolocation, but it can be a helpful tool when you need to bring customers to the market from the street or parking.
While iBeacons with their ability to determine the location of the client with greater accuracy, perform better if you need services based on geolocation indoors. For example, the beacons can be used to provide more accurate navigation inside the building.
Vs Studio Mac App Embedded Resource Locations
Geolocation for IoT
Geofencing and iBeacon evolution have led to a new round of IoT development.
Currently, the Internet of Things is growing fast and changes the industry landscape. It connects more and more devices inside the shop and provides a superior shopping experience.
Experts predict the market increase up to $1.7 trillion by 2020 and about 29.5 billion connected devices in 2020 (compared to 16.9 billion in 2014).
Geolocation and Security
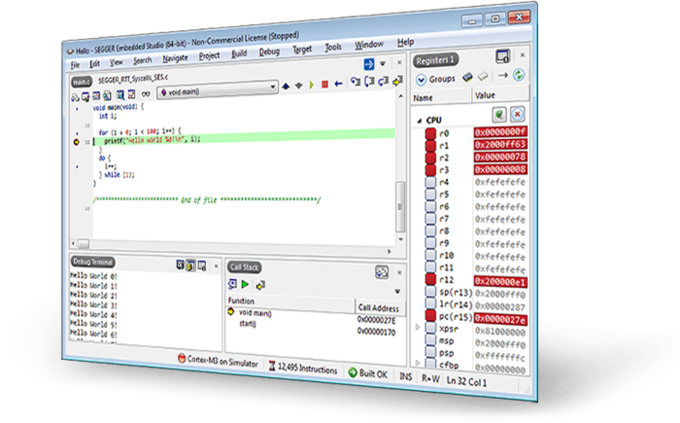
There is a stumbling block, though. Geolocation technologies increase privacy concerns. A vast amount of users refuses to give access to their data as they are an affair that this information could be used to harm them. Companies have to offer valuable benefits to users as well as guarantee information security.
Takeaway
Location-based apps tend to shake up all industries and change the way brands interact with their customers online and offline. Create a geolocation app for your business, and you can always deliver information and benefits to your audience at the right place and time.
Gain trust and ensure customers that their private information is safe - this is the secret ingredient of a successful geolocation app.

Create a GPS app today
What our clients say
Related reading:
Want to receive reading suggestions once a month?
Subscribe to our newsletters
